Texas Holdem Python
Python coding for a game of texas hold em. We are given a starter code, that is given below: import cards def lessthan(c1,c2): 'Return True if c1 is smaller in rank, True if ranks are equal and c1 has a 'smaller' suit False otherwise' if c1.rank. Hi r/learnpython. I've just created a Texas Hold'em implementation in Python and I'd like to share it with you all. Feel free to critique and give feedback, as it was a project to help me get more comfortable with OOP and non flask-based programming. Jun 15, 2020 Texas Hold’em is the most popular variant of poker played today. Many people have started to play casual games with friends as social distancing protocols have adjusted lifestyles. Losing isn’t exactly fun and sometimes the game can seem like it is rigged against you.
pokercards.cards – Represent cards, decks and hands¶
Represents a single french-design card with it’s rank and suit.
Cards can be compared and ordered by rank. A card, relative toa card of the same rank but different suit, is compared as neitherhigher, lower nor equal.
Parameters: |
|
---|---|
Raises : | ValueError |

Create a list of new cards.
Each argument should describe one card with rank and suit together.
Parameters: | args – One or more cards. |
---|---|
Returns: | List of new cards, one for each input parameter. |
Return type: | list of pokercards.cards.Card objects |
Raises : | ValueError |
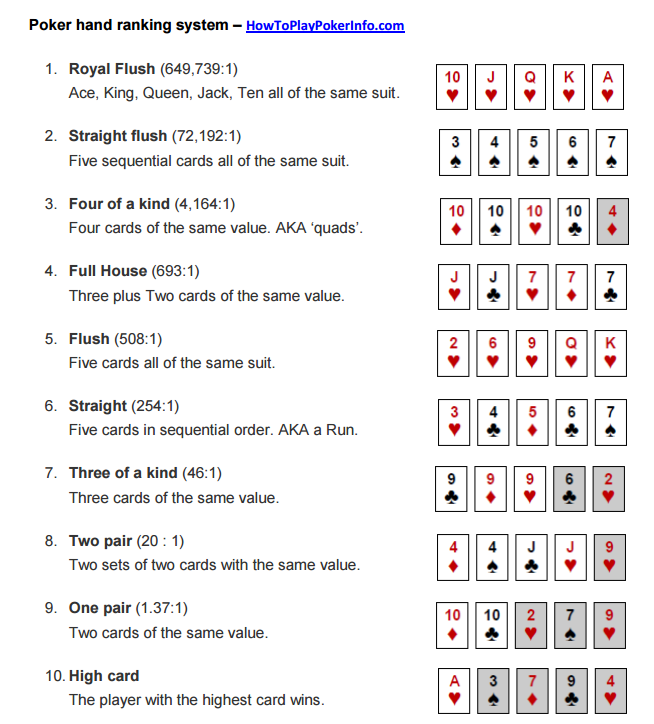
Represents a single deck of 52 card.Card objects.
The deck could be imagined face down on a table. All internal listsrepresent the cards in order from bottom up. So dealing the topcard means poping last item from the list.
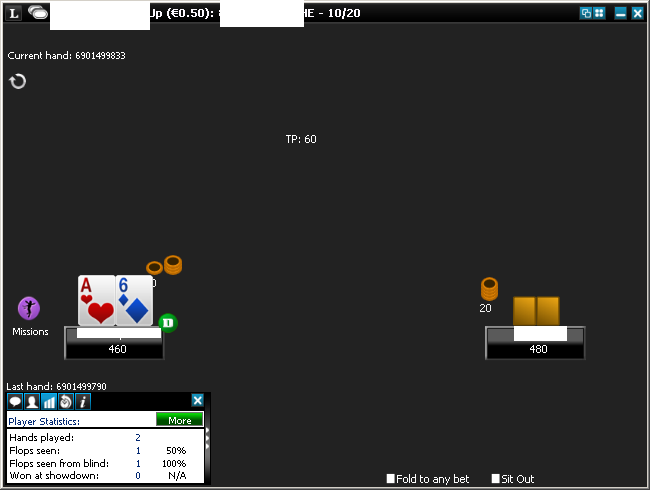
Deal the top card from the deck.
Returns: | pokercards.cards.Card instance |
---|
Shuffle the deck.
Compute the best hand from given cards, implementing traditional“high” poker hand ranks.
The hand object can be given more than five cards (as in TexasHold’em or similar variants) and the evaluation will pick the besthand.

Texas Hold'em Python
Evaluated pokercards.cards.PokerHand objects arecompared and sorted by the rank of the hand.
List of pokercards.cards.Card objects to make the handfrom. The pokercards.cards.PokerHand.evaluate() methodshould be called after manual update to re-evaluate the updatedhand.
Following attributes are available after evaluating the hand.
Readonly rank of the hand (0 = high card to 8 = straight flush)
Readonly list of cards which complete the rank.
Readonly list of extra cards which can break a tie.
Parameters: |
|
---|
Evaluate the rank of the hand.
Should be called either implicitly at start by leavingparameter evaluate True when creating the hand orexplicitly by calling this method later, e.g. after changingthe cards attribute manually.
In this tutorial, you will learn step-by-step how to implement a poker bot in Python.
Step 1 – Setup Python and Install Packages
First, we need an engine in which we can simulate our poker bot. Install the following package (PyPokerEngine) using pip:
It also has a GUI available which can graphically display a game. If you are interested, you can optionally install the following package (PyPokerGUI):
Both the engine and the GUI have excellent tutorials on their GitHub pages in how to use them. The choice for the engine (and/or the GUI) is arbitrary and can be replaced by any engine (and/or GUI) you like. However, the implementation of the bot in this tutorial depends on this choice, so you need to rewrite some if the code if you plan to change the engine (and/or GUI).
Small note on the GUI: it did not work for my directly using Python 3. This fix explains how to make it work.
Step 2 – Implement your Bot!
The first step is to setup the skeleton of the code such that it works. In order to do so, I created three files. One file containing the code for the bot (databloggerbot.py), another file containing the code for a bot which always calls and another file for simulating one game of poker (simulate.py) in which many runs are simulated. The files initially have the following contents:
The bot uses Monte Carlo simulations running from a given state. Suppose you start with 2 high cards (two Kings for example), then the chances are high that you will win. The Monte Carlo simulation then simulates a given number of games from that point and evaluates which percentage of games you will win given these cards. If another King shows during the flop, then your chance of winning will increase. The Monte Carlo simulation starting at that point, will yield a higher winning probability since you will win more games on average.
If we run the simulations, you can see that the bot based on Monte Carlo simulations outperforms the always calling bot. If you start with a stack of $100,-, you will on average end with a stack of $120,- (when playing against the always-calling bot).
It is also possible to play against our bot in the GUI. You first need to setup the configuration (as described in the Git repository) and you can then run the following command to start up the GUI:
Good luck beating the bot!
Texas Hold'em Vegas World
Poker GUI.
Conclusion (TL;DR)
Texas Holdem Python Code
In this simple tutorial, we created a bot based on Monte Carlo simulations. In a later blog post, we will implement a more sophisticated Poker bot using different AI methods. If you have any preference for an AI method, please let me know in the comments!